If you’ve ever wanted to build beautiful, high-performance mobile apps for iOS and Android from a single codebase, Flutter might be the perfect tool for you. Developed by Google, Flutter is an open-source UI toolkit that allows developers to create natively compiled applications not only for mobile but also for web, desktop, and embedded devices.
Whether you’re a seasoned programmer or completely new to app development, this guide will help you understand what Flutter is, why it’s so popular, and how to get started.
What is Flutter?
Flutter is a framework that allows developers to create cross-platform applications using a single programming language, Dart, and a rich set of pre-designed widgets. Unlike traditional cross-platform tools, which rely on bridges to communicate with native components, Flutter renders its UI directly using its own rendering engine. This results in fast, smooth, and highly customizable apps.
Why Use Flutter?
- Cross-Platform Development
With Flutter, you write your code once and deploy it to multiple platforms, including iOS, Android, web, and desktop. This saves time and reduces the effort required to maintain separate codebases. - Fast Development with Hot Reload
Flutter’s hot reload feature allows you to see changes in your app instantly. Modify your code, hit save, and watch the updates appear in real time without restarting the app. - Rich Widget Library
Flutter offers a vast collection of widgets for designing intuitive and beautiful UIs. Widgets can be customized extensively, making it easy to match your app’s unique branding. - High Performance
Since Flutter apps are compiled directly into native machine code, they deliver performance that’s nearly identical to apps written with native frameworks like Swift or Kotlin. - Strong Community and Backing by Google
Flutter is backed by Google and has a thriving global community, which means plenty of learning resources, third-party libraries, and regular updates.
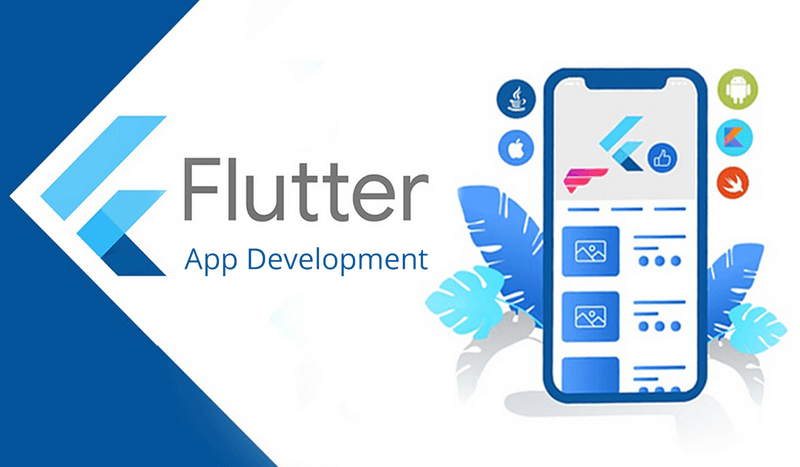
Getting Started with Flutter
1. Install Flutter
To begin, you need to install Flutter on your machine.
- Step 1: Download Flutter SDK
Head to Flutter’s official website and download the SDK for your operating system. - Step 2: Set Up Your Development Environment
Install a code editor like Visual Studio Code or Android Studio, both of which support Flutter development. - Step 3: Add Flutter to Your System Path
Add theflutter/bin
directory to your system’s PATH to use theflutter
command from the terminal. - Step 4: Verify Installation
Runflutter doctor
in your terminal to check if all necessary tools are installed. This command will guide you through installing missing dependencies like Android Studio, Xcode (for iOS development), or additional plugins.
2. Learn the Basics of Dart
Flutter uses Dart, a programming language also developed by Google. It’s similar to JavaScript, making it approachable for beginners. Start by learning:
- Variables and Data Types
- Functions and Classes
- Control Flow (if-else, loops)
- Asynchronous Programming with Futures and Streams
Dart tutorials are readily available on the Dart website.
3. Create Your First Flutter App
- Step 1: Open the Terminal
Run the command:flutter create my_first_app cd my_first_app flutter run
- Step 2: Explore the Starter Code
The default template includes a simple counter app. Openlib/main.dart
to see how Flutter structures its code. - Step 3: Modify the Code
Experiment with changing text, colors, or adding widgets. Use hot reload to instantly view the updates in your app.
4. Understand Flutter’s Core Concepts
- Widgets
Widgets are the building blocks of a Flutter app. Everything in Flutter is a widget, from buttons to entire layouts. Widgets can be:- Stateless: Static content that doesn’t change (e.g., text or icons).
- Stateful: Dynamic content that changes based on user interaction (e.g., forms or animations).
- Material Design and Cupertino
Flutter supports Google’s Material Design for Android and Cupertino widgets for iOS, ensuring platform-specific look and feel. - Layouts
Flutter layouts are created using widgets likeColumn
,Row
, andStack
. These widgets arrange and align other widgets flexibly. - Navigation and Routing
Use theNavigator
widget to handle navigation between screens. For example:Navigator.push(context, MaterialPageRoute(builder: (context) => SecondPage()));
Recommended Resources for Beginners
- Official Documentation: The Flutter documentation is beginner-friendly and comprehensive.
- YouTube Tutorials: Channels like “The Net Ninja” and “Traversy Media” have excellent Flutter tutorials.
- Books: “Flutter for Beginners” by Alessandro Biessek is a great starting point.
- Online Courses: Platforms like Udemy and Coursera offer detailed Flutter courses with hands-on projects.
Beginner-Friendly Flutter Projects
Start small to build confidence. Here are a few project ideas:
- To-Do List App: Create a simple app to add, edit, and delete tasks.
- Weather App: Fetch weather data using APIs and display it in a user-friendly UI.
- Quiz App: Develop a multiple-choice quiz app with a scoring system.
- Personal Portfolio App: Showcase your skills and projects in an interactive mobile app.
Conclusion
Flutter is an excellent framework for beginners and professionals alike, offering the tools to create stunning, high-performance apps across platforms. With its intuitive design, robust documentation, and active community, learning Flutter can be a rewarding experience.
So why wait? Download Flutter, start coding, and bring your app ideas to life!
Have questions or want to share your Flutter journey? Let us know in the comments below!
Leave a Reply